Unvired Cordova SDK
This plugin lets you build apps which connect to Unvired Mobile Platform (UMP).
iOS Requirements
Update your Cocoapods repo before you install the plugin.
$ pod repo update
- Browser Requirements
- After you install the plugin, for Ionic/Angular projects, please add a reference to the following JS files within section of index.html.
<script src="assets/js/sql.js"></script>
<script src="assets/js/kernel.js"></script>
<script src="assets/js/winstore-jscompat.js"></script>
<script src="assets/js/jquery-3.2.1.js"></script>
https://github.com/unvired/cordova-plugin-unvired-sdk/
Stuck on a Cordova issue?
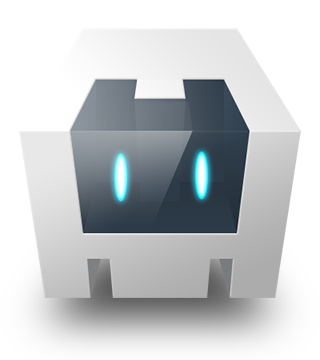
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionicβs experts offer premium advisory services for both community plugins and premier plugins.
Installation
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-unvired-sdk
$ npm install @awesome-cordova-plugins/unvired-cordova-sdk
$ ionic cap sync
$ ionic cordova plugin add cordova-plugin-unvired-sdk
$ npm install @awesome-cordova-plugins/unvired-cordova-sdk
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Β Learn More or if you're interested in an enterprise version of this plugin Contact Us
Supported Platforms
- iOS
- Android
- Windows
- Browser
Usage
React
Learn more about using Ionic Native components in React
Angular
import { UnviredCordovaSDK } from '@awesome-cordova-plugins/unvired-cordova-sdk/ngx';
constructor(private unviredSDK: UnviredCordovaSDK) { }
...
// This is usually done in app.component.ts of your app.
// Before you can interact with UMP, you need to initialize the SDK and authenticate with UMP.
// SDK Initialization
let loginParameters = new LoginParameters()
loginParameters.appName = 'UNVIRED_DIGITAL_FORMS'
loginParameters.metadataPath = '../assets/metadata.json'
let loginResult: LoginResult
try {
loginResult = await this.unviredSDK.login(loginParameters)
}
catch (error) {
this.unviredSDK.logError("AppComponent", "Initialize", "Error during login: " + error)
}
switch (loginResult.type) {
case LoginListenerType.auth_activation_required:
// App is not activated. i.e, User is using the app for the very first time.
// App needs to be activated before it can interact with UMP.
// At this point of time, you basically navigate to a login screen & accept username / password from the user.
// Set the username & password to loginParameters object and call authenticateAndActivate
try {
// Execute this block of code in a login screen.
let loginParameters = new LoginParameters();
loginParameters.url = '<UMP_URL>';
loginParameters.company = '<Company>';
loginParameters.username = '<Username>';
loginParameters.password = '<Password>';
loginParameters.loginType = LoginType.unvired;
let authenticateActivateResult: AuthenticateActivateResult = await this.unviredSDK.authenticateAndActivate(loginParameters);
if (authenticateActivateResult.type === AuthenticateAndActivateResultType.auth_activation_success) {
// App is fully setup. Navigate to your app's home screen.
} else if (authenticateActivateResult.type === AuthenticateAndActivateResultType.auth_activation_error) {
console.log("Error during login: " + authenticateActivateResult.error)
} catch (error) {
this.unviredSDK.logError('LoginPage', 'auth_activation_required', 'ERROR: ' + error);
}
break;
case LoginListenerType.app_requires_login:
// App is already activated. But, the user needs to enter credentials because the setting LOCAL_PASSWORD is set to YES in Unvired Admin Cockpit.
// To set LOCAL_PASSWORD property for your app, contact your administrator.
try {
// Execute this block of code in a login screen.
let loginParameters = new LoginParameters()
loginParameters.username = '<Username>';
loginParameters.password = '<Password>';
let authenticateLocalResult: AuthenticateLocalResult = await this.unviredSDK.authenticateLocal(loginParameters);
if (authenticateLocalResult.type === AuthenticateLocalResultType.login_success) {
// App is fully setup. Navigate to your app's home screen.
} else if (authenticateLocalResult.type === AuthenticateLocalResultType.login_error) {
console.log("Error during local login: " + authenticateActivateResult.error)
} catch (error) {
this.unviredSDK.logError('LoginPage', 'app_requires_login', 'ERROR: ' + error);
}
break;
case login_success:
// The setting LOCAL_PASSWORD is set to false.
// App is fully initialized. Users can interact with the UMP
// Navigate to Home screen
break;
}
// Synchronization APIs
// Example 1: Make a Sync RQST call
await this.unviredSDK.syncForeground(RequestType.RQST, {"CUSTOMER_HEADER": {"CUST_NO" : "007", "CUST_NAME" : "James Bond"}}, '', 'PA_GET_CUSTOMER_DETAILS', true)
// Example 2: Make a Sync QUERY / PULL call
await this.unviredSDK.syncForeground(RequestType.QUERY, '', {"CUSTOMER_SEARCH_CONTEXT":[{"CUSTOMER_SEARCH_HEADER":{"CUST_NO":"007"}}]}, 'PA_SEARCH_CUSTOMER', true)
Example 3: Make a Async RQST call
await this.unviredSDK.syncBackground(RequestType.RQST, {"CUSTOMER_HEADER": {"CUST_NO" : "007", "CUST_NAME" : "James Bond"}}, '', 'PA_GET_CUSTOMER_DETAILS', 'CUSTOMER', beLID, false)
Example 4: Make a Async QUERY / PULL call
await this.unviredSDK.syncBackground(RequestType.QUERY, '', {"CUSTOMER_SEARCH_CONTEXT":[{"CUSTOMER_SEARCH_HEADER":{"CUST_NO":"007"}}]}, 'PA_SEARCH_CUSTOMER', '', '', true)
// Note: Subscribe to NotificationListener to get updates on data processing in background
// However, only one screen can listen to background data updates at any point of time.
this.unviredSDK.registerNotifListener().subscribe( data => {
switch (data.type) {
case NotificationListenerType.dataSend:
break;
case NotificationListenerType.dataChanged:
break;
case NotificationListenerType.dataReceived:
break;
.
.
.
}})
// Database APIs
// Insert a record onto database
this.unviredsdk.dbInsert("CUSTOMER_HEADER", {"NAME":"James Bond","CUST_NO":"007"}, true);
// Update a record in database
this.unviredSDK.dbUpdate('CUSTOMER_HEADER', {"NAME":"John Doe","CUST_NO":"008"}, "CUST_NO = '007'")
// Delete a record in database
this.unviredSDK.dbDelete('CUSTOMER_HEADER', "CUST_NO = '007'")
// Execute a SQL Query
this.unviredSDK.dbExecuteStatement('SELECT * FROM CUSTOMER_HEADER WHERE CUSTOMER_ID = "007"')