3D Touch
The 3D Touch plugin adds 3D Touch capabilities to your Cordova app.
Requires Cordova plugin: cordova-plugin-3dtouch
. For more info, please see the 3D Touch plugin docs.
https://github.com/EddyVerbruggen/cordova-plugin-3dtouch
Stuck on a Cordova issue?
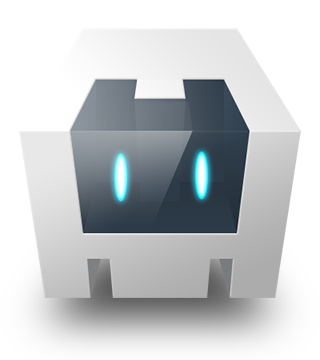
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionicβs experts offer premium advisory services for both community plugins and premier plugins.
Installation
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-3dtouch
$ npm install @awesome-cordova-plugins/three-dee-touch
$ ionic cap sync
$ ionic cordova plugin add cordova-plugin-3dtouch
$ npm install @awesome-cordova-plugins/three-dee-touch
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Β Learn More or if you're interested in an enterprise version of this plugin Contact Us
Supported Platforms
- iOS
Usage
React
Learn more about using Ionic Native components in React
Angular
Please do refer to the original plugin's repo for detailed usage. The usage example here might not be sufficient.
import { ThreeDeeTouch, ThreeDeeTouchQuickAction, ThreeDeeTouchForceTouch } from '@awesome-cordova-plugins/three-dee-touch/ngx';
constructor(private threeDeeTouch: ThreeDeeTouch) { }
...
this.threeDeeTouch.isAvailable().then(isAvailable => console.log('3D Touch available? ' + isAvailable));
this.threeDeeTouch.watchForceTouches()
.subscribe(
(data: ThreeDeeTouchForceTouch) => {
console.log('Force touch %' + data.force);
console.log('Force touch timestamp: ' + data.timestamp);
console.log('Force touch x: ' + data.x);
console.log('Force touch y: ' + data.y);
}
);
let actions: ThreeDeeTouchQuickAction[] = [
{
type: 'checkin',
title: 'Check in',
subtitle: 'Quickly check in',
iconType: 'Compose'
},
{
type: 'share',
title: 'Share',
subtitle: 'Share like you care',
iconType: 'Share'
},
{
type: 'search',
title: 'Search',
iconType: 'Search'
},
{
title: 'Show favorites',
iconTemplate: 'HeartTemplate'
}
];
this.threeDeeTouch.configureQuickActions(actions);
this.threeDeeTouch.onHomeIconPressed().subscribe(
(payload) => {
// returns an object that is the button you presed
console.log('Pressed the ${payload.title} button')
console.log(payload.type)
}
)