Email Composer
Requires Cordova plugin: cordova-plugin-email-composer. For more info, please see the Email Composer plugin docs.
https://github.com/katzer/cordova-plugin-email-composer
Stuck on a Cordova issue?
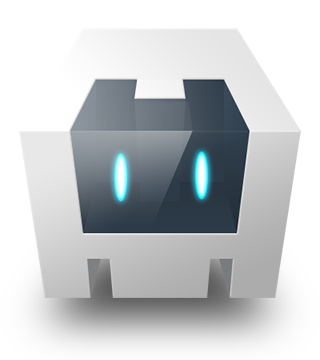
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionicβs experts offer premium advisory services for both community plugins and premier plugins.
Installation
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-email-composer
$ npm install @awesome-cordova-plugins/email-composer
$ ionic cap sync
$ ionic cordova plugin add cordova-plugin-email-composer
$ npm install @awesome-cordova-plugins/email-composer
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Β Learn More or if you're interested in an enterprise version of this plugin Contact Us
Supported Platforms
- Amazon Fire OS
- Android
- Browser
- iOS
- Windows
- macOS
Usage
React
Learn more about using Ionic Native components in React
Angular
import { EmailComposer } from '@awesome-cordova-plugins/email-composer/ngx';
constructor(private emailComposer: EmailComposer) { }
...
this.emailComposer.getClients().then((apps: []) => {
// Returns an array of configured email clients for the device
});
this.emailComposer.hasClient().then(app, (isValid: boolean) => {
if (isValid) {
// Now we know we have a valid email client configured
// Not specifying an app will return true if at least one email client is configured
}
});
this.emailComposer.hasAccount().then((isValid: boolean) => {
if (isValid) {
// Now we know we have a valid email account configured
}
});
this.emailComposer.isAvailable().then(app, (available: boolean) => {
if(available) {
// Now we know we can send an email, calls hasClient and hasAccount
// Not specifying an app will return true if at least one email client is configured
}
});
let email = {
to: 'max@mustermann.de',
cc: 'erika@mustermann.de',
bcc: ['john@doe.com', 'jane@doe.com'],
attachments: [
'file://img/logo.png',
'res://icon.png',
'base64:icon.png//iVBORw0KGgoAAAANSUhEUg...',
'file://README.pdf'
],
subject: 'Cordova Icons',
body: 'How are you? Nice greetings from Leipzig',
isHtml: true
}
// Send a text message using default options
this.emailComposer.open(email);
You can also assign aliases to email apps
// add alias
this.email.addAlias('gmail', 'com.google.android.gm');
// then use alias when sending email
this.email.open({
app: 'gmail',
...
});