File Transfer
This plugin allows you to upload and download files.
https://github.com/apache/cordova-plugin-file-transfer
Stuck on a Cordova issue?
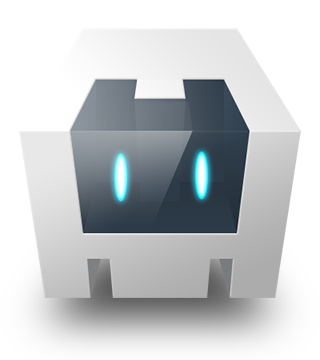
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionicβs experts offer premium advisory services for both community plugins and premier plugins.
Installation
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-file-transfer
$ npm install @awesome-cordova-plugins/file-transfer
$ ionic cap sync
$ ionic cordova plugin add cordova-plugin-file-transfer
$ npm install @awesome-cordova-plugins/file-transfer
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Β Learn More or if you're interested in an enterprise version of this plugin Contact Us
Supported Platforms
- Amazon Fire OS
- Android
- Browser
- iOS
- Ubuntu
- Windows
- Windows Phone
Usage
React
Learn more about using Ionic Native components in React
Angular
import { FileTransfer, FileUploadOptions, FileTransferObject } from '@awesome-cordova-plugins/file-transfer/ngx';
import { File } from '@awesome-cordova-plugins/file';
constructor(private transfer: FileTransfer, private file: File) { }
...
const fileTransfer: FileTransferObject = this.transfer.create();
// Upload a file:
fileTransfer.upload(..).then(..).catch(..);
// Download a file:
fileTransfer.download(..).then(..).catch(..);
// Abort active transfer:
fileTransfer.abort();
// full example
upload() {
let options: FileUploadOptions = {
fileKey: 'file',
fileName: 'name.jpg',
headers: {}
.....
}
fileTransfer.upload('<file path>', '<api endpoint>', options)
.then((data) => {
// success
}, (err) => {
// error
})
}
download() {
const url = 'http://www.example.com/file.pdf';
fileTransfer.download(url, this.file.dataDirectory + 'file.pdf').then((entry) => {
console.log('download complete: ' + entry.toURL());
}, (error) => {
// handle error
});
}
To store files in a different/publicly accessible directory, please refer to the following link https://github.com/apache/cordova-plugin-file#where-to-store-files