AES256
This cordova ionic plugin allows you to perform AES 256 encryption and decryption on the plain text. It's a cross-platform plugin which supports both Android and iOS. The encryption and decryption are performed on the device native layer so that the performance is much faster.
https://github.com/Ideas2IT/cordova-aes256
Stuck on a Cordova issue?
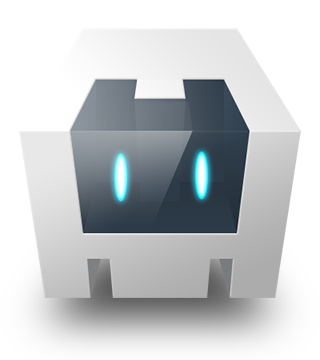
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionicβs experts offer premium advisory services for both community plugins and premier plugins.
Installation
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-aes256-encryption
$ npm install @awesome-cordova-plugins/aes-256
$ ionic cap sync
$ ionic cordova plugin add cordova-plugin-aes256-encryption
$ npm install @awesome-cordova-plugins/aes-256
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Β Learn More or if you're interested in an enterprise version of this plugin Contact Us
Supported Platforms
- Android
- iOS
Usage
React
Learn more about using Ionic Native components in React
Angular
import { AES256 } from '@awesome-cordova-plugins/aes-256/ngx';
private secureKey: string;
private secureIV: string;
constructor(private aes256: AES256) {
this.generateSecureKeyAndIV(); // To generate the random secureKey and secureIV
}
...
async generateSecureKeyAndIV() {
this.secureKey = await this.aes256.generateSecureKey('random password 12345'); // Returns a 32 bytes string
this.secureIV = await this.aes256.generateSecureIV('random password 12345'); // Returns a 16 bytes string
}
this.aes256.encrypt(this.secureKey, this.secureIV, 'testdata')
.then(res => console.log('Encrypted Data: ',res))
.catch((error: any) => console.error(error));
this.aes256.decrypt(this.secureKey, this.secureIV, 'encryptedData')
.then(res => console.log('Decrypted Data : ',res))
.catch((error: any) => console.error(error));
* this.aes256.generateSecureKey('random password 12345')
.then(res => console.log('Secure Key : ',res))
.catch((error: any) => console.error(error));
* this.aes256.generateSecureIV('random password 12345')
.then(res => console.log('Secure IV : ',res))
.catch((error: any) => console.error(error));